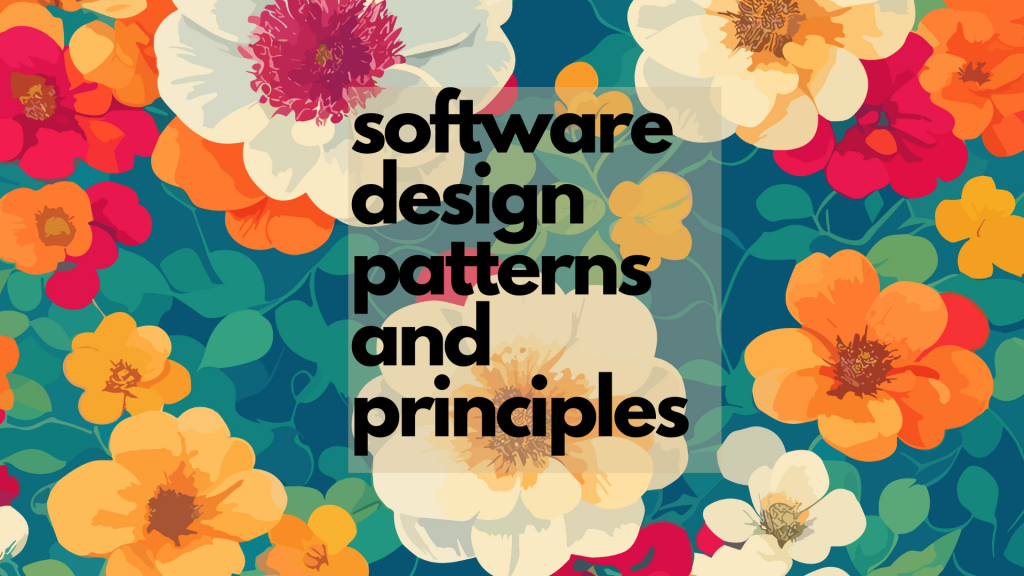
When architecting a software system, using proven design patterns can help create more robust, flexible, and maintainable code. Here are some key principles and patterns to follow:
Break Programs into Logical Components Decompose software into self-contained components with clear responsibilities. Allow necessary dependencies between components, but limit coupling. This aids reusability and independent development.
Simplify Component Interactions Inspect data flows between components. Keep classes small with single responsibilities. Avoid complex webs of components by enforcing simplified one-way data flows where possible.
Limit Logic in Data Models Data model objects should represent state and data, not control logic. Keep business logic in controllers and services. This reduces coupling and complexity.
Follow Composition Over Inheritance Favor object composition rather than tight hierarchical inheritance relationships. Composition allows more flexibility when refactoring code.
Carefully Manage Singletons Singletons can simplify independent flows but can also lead to hidden coupling. Use dependency injection to “scope” singletons and improve testability.
Implement Common Communication Patterns Publisher-subscriber, delegates, and chain of responsibility are common communication patterns between components that avoid tight coupling.
Use Common Creational Patterns Builder classes, factories, and lazy initialization can simplify complex object creation logic and improve performance.
There are many other structural, behavioral, and creational patterns that solve common code challenges. Learning design patterns builds intuition for crafting more intentional, evolvable software systems. Mastering design principles takes practice – but the payoff is code that can stand the test of time.
Single Responsibility Principle
- Each class and module should have one clear purpose or responsibility.
- Example: A UserService class for user management vs UserValidation for input checking.
Open/Closed Principle
- Code should be open for extension but closed for modification.
- Example: Allow new Report types by creating Report subclasses rather than modifying a Report class.
Composition Over Inheritance
- Favor composition of objects instead of tight class hierarchies.
- Example: A Car class can contain a Motor object instead of subclassing MotorVehicle.
Dependency Inversion
- Depend on abstractions rather than concrete implementations. Use interfaces and dependency injection.
- Example: Code relies on a PersistenceInterface rather than a SQLDatabase class.
Observer Pattern
- Components subscribe to an event system and get notified of changes.
- Example: UI elements subscribe to data model changes to update.
Decorator Pattern
- Dynamically add behaviors to objects by wrapping them.
- Example: Wrap a Message class with encryption and compression decorators.
Factory Pattern
- Encapsulate complex object creation logic in a factory class.
- Example: ShapeFactory handles creating Circles, Squares etc.
Facade Pattern
- Provide a simple interface to a complex subsystem.
- Example: FileManager facade hides lower-level filesystem details.